Welcome to Software Development on Codidact!
Will you help us build our independent community of developers helping developers? We're small and trying to grow. We welcome questions about all aspects of software development, from design to code to QA and more. Got questions? Got answers? Got code you'd like someone to review? Please join us.
Post History
I was wondering if somebody can review my code? I am creating a simple login desktop application, just to get used to the MVVM pattern using WPF. View <Window x:Class="Login_App.MainWindow" ...
#2: Post edited
- I was wondering if somebody can review my code? I am creating a simple login desktop application, just to get used to the MVVM pattern using WPF.
- **View**
- <Window x:Class="Login_App.MainWindow"
- xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
- xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
- xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
- xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
- xmlns:local="clr-namespace:Login_App"
- xmlns:vm ="clr-namespace:Login_App.ViewModel"
- mc:Ignorable="d"
- Title="Login" Height="380" Width="650" ResizeMode="NoResize" WindowStartupLocation="CenterScreen">
- <Window.Resources>
- <ResourceDictionary>
- <vm:LoginVM x:Key="vm"/>
- </ResourceDictionary>
- </Window.Resources>
- <StackPanel DataContext="{StaticResource vm}">
- <TextBlock Text="Username"/>
- <TextBox Text="{Binding Username, Mode=TwoWay, UpdateSourceTrigger=PropertyChanged}"/>
- <TextBlock Text="Password"/>
- <TextBox Text="{Binding Password, Mode=TwoWay, UpdateSourceTrigger=PropertyChanged}"/>
- <Button Content="Login"
- Command="{Binding LoginCommand}"
- CommandParameter="{Binding User}"/>
- </StackPanel>
- </Window>
- **LoginView.xaml.cs**
- namespace Login_App
- {
- /// <summary>
- /// Interaction logic for MainWindow.xaml
- /// </summary>
- public partial class MainWindow : Window
- {
- LoginVM viewModel;
- public MainWindow()
- {
- InitializeComponent();
- viewModel = Resources["vm"] as LoginVM;
- viewModel.Authenticated += ViewModel_Authenticated;
- }
- private void ViewModel_Authenticated(object sender, EventArgs e)
- {
- Close();
- }
- }
- }
- **Model**
- namespace Login_App.Model
- {
- public class UserModel
- {
- public int UserID { get; set; }
- public string Username { get; set; }
- public string Email { get; set; }
- public string Password { get; set; }
- public int DepartmentID { get; set; }
- public int GroupID { get; set; }
- public bool Inactive { get; set; }
- }
- }
- **ViewModel**
- This is the structure:
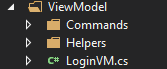- **LoginVM.cs**
- namespace Login_App.ViewModel
- {
- public class LoginVM : INotifyPropertyChanged
- {
- private UserModel user;
- public UserModel User
- {
- get { return user; }
- set
- {
- user = value;
- OnPropertyChanged("User");
- }
- }
- private string username;
- public string Username
- {
- get { return username; }
- set
- {
- username = value;
- User = new UserModel
- {
- Username = username,
- Password = this.Password
- };
- OnPropertyChanged("Username");
- }
- }
- private string password;
- public string Password
- {
- get { return password; }
- set
- {
- password = value;
- User = new UserModel
- {
- Username = this.Username,
- Password = password
- };
- OnPropertyChanged("Password");
- }
- }
- public Commands.LoginCommand LoginCommand { get; set; }
- public LoginVM()
- {
- LoginCommand = new Commands.LoginCommand(this);
- user = new UserModel();
- }
- public async void Login()
- {
- bool result = await Helpers.MySQLHelper.Login(User);
- if (result)
- {
- Authenticated?.Invoke(this, new EventArgs());
- }
- }
- public EventHandler Authenticated;
- public event PropertyChangedEventHandler PropertyChanged;
- private void OnPropertyChanged(string propertyName)
- {
- PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
- }
- }
- }
- **Commands > LoginCommand.cs**
- namespace Login_App.ViewModel.Commands
- {
- public class LoginCommand : ICommand
- {
- public LoginVM ViewModel { get; set; }
- public event EventHandler CanExecuteChanged
- {
- add { CommandManager.RequerySuggested += value; }
- remove { CommandManager.RequerySuggested -= value; }
- }
- public LoginCommand(LoginVM vm)
- {
- ViewModel = vm;
- }
- public bool CanExecute(object parameter)
- {
- UserModel user = parameter as UserModel;
- if (user == null)
- return false;
- if (string.IsNullOrEmpty(user.Username))
- return false;
- if (string.IsNullOrEmpty(user.Password))
- return false;
- return true;
- }
- public void Execute(object parameter)
- {
- ViewModel.Login();
- }
- }
- }
- **Helpers > MySQLHelper.cs**
- public class MySQLHelper
- {
- // Login Functionality
- public static async Task<bool> Login(UserModel user)
- {
- try
- {
- /// Query to get count of users from the parameters provided by the user.
- /// On successful Login, the details from the database,
- /// will be saved in the <App.xaml.cs> file.
- string mysqlUserDetails = "SELECT COUNT(*), u.id AS UserID, Username, Email, DepartmentID, Inactive" +
- " FROM users u WHERE Username = @Username AND Password = MD5(@Password)";
- using(var connection = new MySqlConnection(ConfigurationManager.ConnectionStrings["MySQL"].ConnectionString))
- {
- var userDetails = connection.Query<UserModel>(mysqlUserDetails, new { Username = user.Username, Password = user.Password }).ToList();
- // Determine whether to autherize or not
- if(userDetails.Count == 1)
- {
- var details = userDetails.First();
- App.UserId = details.UserID;
- App.Username = details.Username;
- App.Email = details.Email;
- App.DepartmentID = details.DepartmentID;
- App.Inative = details.Inactive;
- }
- }
- return false;
- }
- catch (Exception ex)
- {
- return false;
- }
- }
- }
- I was wondering if somebody can review my code? I am creating a simple login desktop application, just to get used to the MVVM pattern using WPF.
- **View**
- <Window x:Class="Login_App.MainWindow"
- xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
- xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
- xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
- xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
- xmlns:local="clr-namespace:Login_App"
- xmlns:vm ="clr-namespace:Login_App.ViewModel"
- mc:Ignorable="d"
- Title="Login" Height="380" Width="650" ResizeMode="NoResize" WindowStartupLocation="CenterScreen">
- <Window.Resources>
- <ResourceDictionary>
- <vm:LoginVM x:Key="vm"/>
- </ResourceDictionary>
- </Window.Resources>
- <StackPanel DataContext="{StaticResource vm}">
- <TextBlock Text="Username"/>
- <TextBox Text="{Binding Username, Mode=TwoWay, UpdateSourceTrigger=PropertyChanged}"/>
- <TextBlock Text="Password"/>
- <TextBox Text="{Binding Password, Mode=TwoWay, UpdateSourceTrigger=PropertyChanged}"/>
- <Button Content="Login"
- Command="{Binding LoginCommand}"
- CommandParameter="{Binding User}"/>
- </StackPanel>
- </Window>
- **LoginView.xaml.cs**
- namespace Login_App
- {
- /// <summary>
- /// Interaction logic for MainWindow.xaml
- /// </summary>
- public partial class MainWindow : Window
- {
- LoginVM viewModel;
- public MainWindow()
- {
- InitializeComponent();
- viewModel = Resources["vm"] as LoginVM;
- viewModel.Authenticated += ViewModel_Authenticated;
- }
- private void ViewModel_Authenticated(object sender, EventArgs e)
- {
- Close();
- }
- }
- }
- **Model**
- namespace Login_App.Model
- {
- public class UserModel
- {
- public int UserID { get; set; }
- public string Username { get; set; }
- public string Email { get; set; }
- public string Password { get; set; }
- public int DepartmentID { get; set; }
- public int GroupID { get; set; }
- public bool Inactive { get; set; }
- }
- }
- **ViewModel**
- This is the structure:
- 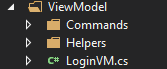
- **LoginVM.cs**
- namespace Login_App.ViewModel
- {
- public class LoginVM : INotifyPropertyChanged
- {
- private UserModel user;
- public UserModel User
- {
- get { return user; }
- set
- {
- user = value;
- OnPropertyChanged("User");
- }
- }
- private string username;
- public string Username
- {
- get { return username; }
- set
- {
- username = value;
- User = new UserModel
- {
- Username = username,
- Password = this.Password
- };
- OnPropertyChanged("Username");
- }
- }
- private string password;
- public string Password
- {
- get { return password; }
- set
- {
- password = value;
- User = new UserModel
- {
- Username = this.Username,
- Password = password
- };
- OnPropertyChanged("Password");
- }
- }
- public Commands.LoginCommand LoginCommand { get; set; }
- public LoginVM()
- {
- LoginCommand = new Commands.LoginCommand(this);
- user = new UserModel();
- }
- public async void Login()
- {
- bool result = await Helpers.MySQLHelper.Login(User);
- if (result)
- {
- Authenticated?.Invoke(this, new EventArgs());
- }
- }
- public EventHandler Authenticated;
- public event PropertyChangedEventHandler PropertyChanged;
- private void OnPropertyChanged(string propertyName)
- {
- PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
- }
- }
- }
- **Commands > LoginCommand.cs**
- namespace Login_App.ViewModel.Commands
- {
- public class LoginCommand : ICommand
- {
- public LoginVM ViewModel { get; set; }
- public event EventHandler CanExecuteChanged
- {
- add { CommandManager.RequerySuggested += value; }
- remove { CommandManager.RequerySuggested -= value; }
- }
- public LoginCommand(LoginVM vm)
- {
- ViewModel = vm;
- }
- public bool CanExecute(object parameter)
- {
- UserModel user = parameter as UserModel;
- if (user == null)
- return false;
- if (string.IsNullOrEmpty(user.Username))
- return false;
- if (string.IsNullOrEmpty(user.Password))
- return false;
- return true;
- }
- public void Execute(object parameter)
- {
- ViewModel.Login();
- }
- }
- }
- **Helpers > MySQLHelper.cs**
- public class MySQLHelper
- {
- // Login Functionality
- public static async Task<bool> Login(UserModel user)
- {
- try
- {
- /// Query to get count of users from the parameters provided by the user.
- /// On successful Login, the details from the database,
- /// will be saved in the <App.xaml.cs> file.
- string mysqlUserDetails = "SELECT COUNT(*), u.id AS UserID, Username, Email, DepartmentID, Inactive" +
- " FROM users u WHERE Username = @Username AND Password = MD5(@Password)";
- using(var connection = new MySqlConnection(ConfigurationManager.ConnectionStrings["MySQL"].ConnectionString))
- {
- var userDetails = connection.Query<UserModel>(mysqlUserDetails, new { Username = user.Username, Password = user.Password }).ToList();
- // Determine whether to autherize or not
- if(userDetails.Count == 1)
- {
- var details = userDetails.First();
- App.UserId = details.UserID;
- App.Username = details.Username;
- App.Email = details.Email;
- App.DepartmentID = details.DepartmentID;
- App.Inative = details.Inactive;
- }
- }
- return false;
- }
- catch (Exception ex)
- {
- return false;
- }
- }
- }
#1: Initial revision
C# MVVM Login Project
I was wondering if somebody can review my code? I am creating a simple login desktop application, just to get used to the MVVM pattern using WPF. **View** <Window x:Class="Login_App.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:local="clr-namespace:Login_App" xmlns:vm ="clr-namespace:Login_App.ViewModel" mc:Ignorable="d" Title="Login" Height="380" Width="650" ResizeMode="NoResize" WindowStartupLocation="CenterScreen"> <Window.Resources> <ResourceDictionary> <vm:LoginVM x:Key="vm"/> </ResourceDictionary> </Window.Resources> <StackPanel DataContext="{StaticResource vm}"> <TextBlock Text="Username"/> <TextBox Text="{Binding Username, Mode=TwoWay, UpdateSourceTrigger=PropertyChanged}"/> <TextBlock Text="Password"/> <TextBox Text="{Binding Password, Mode=TwoWay, UpdateSourceTrigger=PropertyChanged}"/> <Button Content="Login" Command="{Binding LoginCommand}" CommandParameter="{Binding User}"/> </StackPanel> </Window> **LoginView.xaml.cs** namespace Login_App { /// <summary> /// Interaction logic for MainWindow.xaml /// </summary> public partial class MainWindow : Window { LoginVM viewModel; public MainWindow() { InitializeComponent(); viewModel = Resources["vm"] as LoginVM; viewModel.Authenticated += ViewModel_Authenticated; } private void ViewModel_Authenticated(object sender, EventArgs e) { Close(); } } } **Model** namespace Login_App.Model { public class UserModel { public int UserID { get; set; } public string Username { get; set; } public string Email { get; set; } public string Password { get; set; } public int DepartmentID { get; set; } public int GroupID { get; set; } public bool Inactive { get; set; } } } **ViewModel** This is the structure: ![Image alt text] (https://software.codidact.com/uploads/hZBBnKbxeQGABvZGgVWGkcvu) **LoginVM.cs** namespace Login_App.ViewModel { public class LoginVM : INotifyPropertyChanged { private UserModel user; public UserModel User { get { return user; } set { user = value; OnPropertyChanged("User"); } } private string username; public string Username { get { return username; } set { username = value; User = new UserModel { Username = username, Password = this.Password }; OnPropertyChanged("Username"); } } private string password; public string Password { get { return password; } set { password = value; User = new UserModel { Username = this.Username, Password = password }; OnPropertyChanged("Password"); } } public Commands.LoginCommand LoginCommand { get; set; } public LoginVM() { LoginCommand = new Commands.LoginCommand(this); user = new UserModel(); } public async void Login() { bool result = await Helpers.MySQLHelper.Login(User); if (result) { Authenticated?.Invoke(this, new EventArgs()); } } public EventHandler Authenticated; public event PropertyChangedEventHandler PropertyChanged; private void OnPropertyChanged(string propertyName) { PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName)); } } } **Commands > LoginCommand.cs** namespace Login_App.ViewModel.Commands { public class LoginCommand : ICommand { public LoginVM ViewModel { get; set; } public event EventHandler CanExecuteChanged { add { CommandManager.RequerySuggested += value; } remove { CommandManager.RequerySuggested -= value; } } public LoginCommand(LoginVM vm) { ViewModel = vm; } public bool CanExecute(object parameter) { UserModel user = parameter as UserModel; if (user == null) return false; if (string.IsNullOrEmpty(user.Username)) return false; if (string.IsNullOrEmpty(user.Password)) return false; return true; } public void Execute(object parameter) { ViewModel.Login(); } } } **Helpers > MySQLHelper.cs** public class MySQLHelper { // Login Functionality public static async Task<bool> Login(UserModel user) { try { /// Query to get count of users from the parameters provided by the user. /// On successful Login, the details from the database, /// will be saved in the <App.xaml.cs> file. string mysqlUserDetails = "SELECT COUNT(*), u.id AS UserID, Username, Email, DepartmentID, Inactive" + " FROM users u WHERE Username = @Username AND Password = MD5(@Password)"; using(var connection = new MySqlConnection(ConfigurationManager.ConnectionStrings["MySQL"].ConnectionString)) { var userDetails = connection.Query<UserModel>(mysqlUserDetails, new { Username = user.Username, Password = user.Password }).ToList(); // Determine whether to autherize or not if(userDetails.Count == 1) { var details = userDetails.First(); App.UserId = details.UserID; App.Username = details.Username; App.Email = details.Email; App.DepartmentID = details.DepartmentID; App.Inative = details.Inactive; } } return false; } catch (Exception ex) { return false; } } }